Sign in to follow this
Followers
0
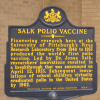
[KotOR] Detect actor setting trap
By
Salk, in General Kotor/TSL Modding
By
Salk, in General Kotor/TSL Modding
By using this site, you agree to our Guidelines.